a cheat sheet for the terminal (macOS command-line interface)
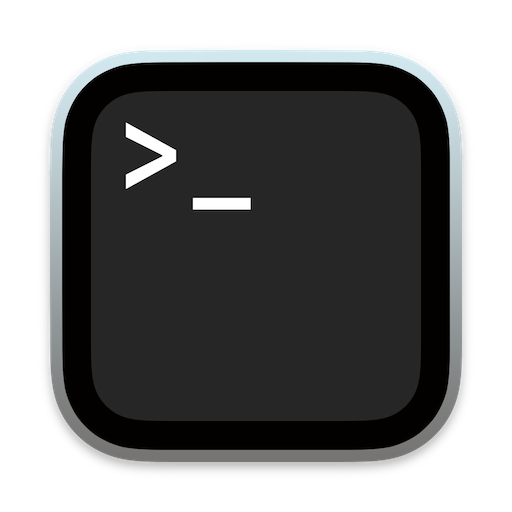
The Terminal is a quick and powerful way to accomplish tasks on your computer, and it is essential for working with development environments like python and node. Before working with these languages, having a basic knowledge of Unix shell is reccommended. This post is a list of common commands for dealing with the command-line.
navigating directories šļø
File management through the command-line can be a little bit less intuitive than using a GUI (graphical user interface) browser like the Finder, but it does allow you to utilize your files and folders in scripts. You can just refer to them by their paths. Below are the most fundamental commands you'll be using for moving through your folders.
print your current working directory
pwd
list files
ls
change directory
cd relative/path # or cd ~/absolute/path
move up one directory
cd ..
move up two directories and so on...
cd ../..
go back to your previous directory
cd -
change directory, but add the path to a directory stack (a history of directories)
pushd folder/path
go back one directory in the stack while also removing the directory you're leaving from the stack
popd
go back one directory in the stack (keeping the stack intact)
pushd +1
go to the bottom of the directory stack
pushd -0
view your directory stack
dirs -v
creating, reading, updating, and deleting āļø
A basic list of common operations that most users carry out through the Finder, like move, copy, delete, etc. Keep in mind, from the perspective of somebody who uses a GUI, a lot of these commands serve dual purposes. For example, you will be using the same command, mv
, to either rename a file or move it.
create a folder
mkdir foldername
create a folder and the necessary parent folders
mkdir -p parentname/foldername
print to the console
echo "hello, world!"
create a file
touch "filename.txt"
direct output towards a file (overwrite)
echo "hello, world!" > filename.txt
direct output towards a file (append)
echo "hello, world!" >> filename.txt
move a file or folder
mv "original/path/to/folder" "new/path/to/folder"
rename a file or folder
mv "orignalName.txt" "newName.txt"
copy a file
cp "original.txt" "copy.txt"
copy a folder
cp -r "original" "copy or destination"
(if the folder "copy or destination"
already exists, the command will place your copy inside of "copy or destination"
)
hide a file or folder
chflags hidden path/to/file
reveal a hidden file or folder
chflags nohidden path/to/file
compare files and/or folders
diff file_a.txt file_b.txt
useful tips š
command history
press ā/ā on your terminal to go through a history of your commands you've used during this session
kill process in the current session
stop a process by pressing ā Control+ C
open a file, folder or application
open path/to/item
development & scripting š»
I find myself using these commands often when running processes or scripting. Retrieving the hostname of your machine can be useful for setting up configuration files for scripts that run on different machines. The IP address of your development machine is often needed when testing locally hosted projects on other devices.
make a script executable
chmod 755 YourScriptName.sh
kill a PID using
kill -9 PID <pid>
Assign
sudo chown -R $(whoami) /usr/local/*
get the PID of a process running on a port
lsof -i tcp:<portnumber>
get the name of your machine
hostname -s
get your machines local IP address using:
# wifi ipconfig getifaddr en1 # ethernet ipconfig getifaddr en0
if you're on an older OS, and the above two don't work, try using
ifconfig | grep "inet " | grep -Fv 127.0.0.1 | awk '{print $2}'
Below is a useful selection of commands for dealing with git, the most popular version control system. Using git
you can log the changes to your work incrementally, in a highly detailed and selective manner, while also using github to store backups of your repositories.
clone a repository
git clone https://github.com/<username>/<repo>
initialize a repository in a directory
git init
check the status of your repo
git status
add a file to your staging area
git add path/to/file
add new & modified files to your staging area
git add .
remove all files from the staging area
git reset
revert your project to your previous commit
git restore .
compare your changes to your previous commit
git diff
configure access to a remote repository
git remote set-url origin https://<your-personal-token>@github.com/<username>/<repo>.git
The above command will initialize your credentials for a repo already on your computer. If you need to clone the repository first, you will have to apply your access token to the clone command in a similar way:
git clone https://<your-personal-token>@github.com/<username>/<repo>.git
macOS settings š„ļø
hide all desktop icons
defaults write com.apple.finder CreateDesktop false # use 'true' instead of 'false' to reveal your hidden desktop icons killall Finder # reset the Finder to take effect
speed up Time Machine backups
sudo sysctl debug.lowpri_throttle_enabled=0
set Time Machine back to it's normal background throttled speed
sudo sysctl debug.lowpri_throttle_enabled=1
keep your mac from falling asleep after disconnecting a screen share session
sudo defaults write /Library/Preferences/com.apple.RemoteManagement RestoreMachineState -bool NO
helpful links š
dev.to - rpalo - bash brackets quick reference youtube.com - Programming with Moshi - Git Tutorial for Beginners: Learn Git in 1 Hour
Published on: April 4, 2024
Edited on: November 27, 2024